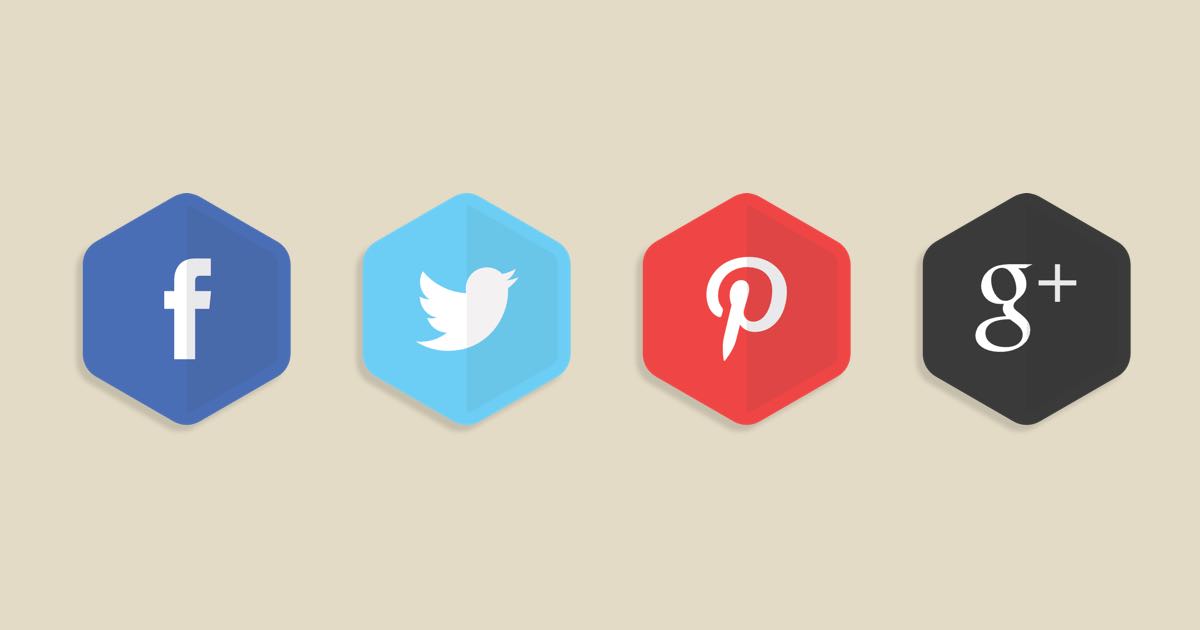
Every major social network has an embeddable share button that you can easily place into a WordPress theme by simply copying and pasting the code from their site. While the ease of use is a plus, embedding these buttons comes with some serious drawbacks. Most importantly, these buttons each load multiple HTTP requests – not such a big deal on a home wifi network, but something that can drastically slow down a site when it’s accessed over a cellular network. In addition, you lose all control over the button appearance and it can be challenging to get them to play nicely together. What’s the solution? Take advantage of the ability to build your own social share links and follow this guide to build an in-post widget for WordPress.
Building Social Share Links
The first step is to learn how these social share links are put together. I’ll walk you through the details of building a Twitter share link as an example, and provide the framework for many other networks towards the end of this tutorial. You can find detailed information available for all networks – here is the Twitter documentation.
Building a Twitter share link is super easy. First, you’ll need to start with the URL base:
https://twitter.com/intent/tweet
Then, you’ll need to add the content of the tweet by utilizing URL parameters. Start with the body of the tweet – perhaps a great quote from the post you’ve just written or maybe the title. You’ll need to make sure that the content is URL encoded. I’ll show you how to do so with PHP later, but for now just use a tool like this. Then use the ‘text’ url parameter to add your content:
https://twitter.com/intent/tweet?text=An%20Optimized%20WordPress%20Widget%20for%20Social%20Share%20Links
Adding that text parameter is all that’s required to make the share link work – click on that link and you’ll see a pre-populated tweet with the title of this post. Of course, it’s not a very helpful tweet. There’s no mention of my username OR the post URL. Luckily, there are a variety of other parameters available (you can see them all in the documentation). Here’s a more useful Twitter share link which takes advantage of the ‘url’ and ‘via’ parameters.
https://twitter.com/intent/tweet?text=An%20Optimized%20WordPress%20Widget%20for%20Social%20Share%20Links&url=https%3A%2F%2Fwww.hweaver.com%2Foptimized-wordpress-widget-social-share-links%2F&via=heatherthedev
So you can see the concept is quite simple. Now it’s just a matter of automatically populating those share links with post data and turning it all into a shortcode for repeat use throughout the site.
Creating a Social Share Widget for WordPress
The bulk of this tutorial will be dedicated to creating a shortcode-based social share link collection that you can embed within the content of any post (or utilize in any part of your custom template), like so:
We want to make this available as a shortcode, so let’s start by opening up functions.php and adding the structure for including a custom shortcode:
function social_share_links_shortcode() { }
add_shortcode('social-share-links', 'social_share_links_shortcode');
With this code, you’ll be able to simply add [social-share-links] to any post or page and make your share links appear at that location.
Unfortunately, right now this doesn’t add anything to the page – we’ll have to build the code which echos the markup to the page. First, let’s start by building the share links. I’ll be setting the following default values for use in various links. Remember to make sure all values are URL encoded (a built-in PHP function).
$title = urlencode(get_the_title());
$url = urlencode(get_the_permalink());
$twitterName = 'heatherthedev';
Now, you can build your links. Head to the next section for guides to various popular social media sites. I’m going to be including links for Twitter, LinkedIn and Facebook, but you can add as many or as few as you like based on this framework.
$twitterLink="https://twitter.com/intent/tweet?text=$title&url=$url&via=$twitterName";
$facebookLink = "https://www.facebook.com/sharer/sharer.php?u=$url";
$linkedinLink = "https://www.linkedin.com/shareArticle?mini=true&url=$url&title=$title";
Now that your links are set, it’s time to use them to build your markup. I’m using FontAwesome to provide the social media icons. I enjoy FontAwesome as all the major social media icons are available, they’re easy to customize, it’s hosted on a CDN and it’s very popular so it’s widely cached on people’s browsers. Here’s the markup-generating PHP:
$returnString = '<div class="social-share-link-container">';
$returnString = $returnString . '<ul>';
$returnString = $returnString . '<li class="title">Share Post:</li>';
$returnString = $returnString . "<li class='link twitter'><a href='$twitterLink' alt='Share $title on Twitter'><i class='fa fa-twitter'></i></a></li>";
$returnString = $returnString . "<li class='link linkedin'><a href='$linkedinLink' alt='Share $title on LinkedIn'><i class='fa fa-linkedin'></i></a></li>";
$returnString = $returnString . "<li class='link facebook'><a href='$facebookLink' alt='Share $title on Facebook'><i class='fa fa-facebook'></i></a></li>";
$returnString = $returnString . '</ul></div>';
return $returnString;
So the complete PHP code is:
function social_share_links_shortcode() {
$title = urlencode(the_title());
$url = urlencode(the_permalink());
$twitterName = 'heatherthedev'; $twitterLink="https://twitter.com/intent/tweet?text=$title&url=$url&via=$twitterName";
$facebookLink = "https://www.facebook.com/sharer/sharer.php?u=$url";
$linkedinLink = "https://www.linkedin.com/shareArticle?mini=true&url=$url&title=$title";
$returnString = '<div class="social-share-link-container">';
$returnString = $returnString . '<ul>';
$returnString = $returnString . '<li class="title">Share Post:</li>';
$returnString = $returnString . "<li class='link twitter'><a href='$twitterLink' alt='Share $title on Twitter'><i class='fa fa-twitter'></i></a></li>";
$returnString = $returnString . "<li class='link linkedin'><a href='$linkedinLink' alt='Share $title on LinkedIn'><i class='fa fa-linkedin'></i></a></li>";
$returnString = $returnString . "<li class='link facebook'><a href='$facebookLink' alt='Share $title on Facebook'><i class='fa fa-facebook'></i></a></li>";
$returnString = $returnString . '</ul></div>';
return $returnString; }
add_shortcode('social-share-links', 'social_share_links_shortcode');
That’s all you need to get your basic HTML and working links into your post. Now all you have to do is add [social-share-links] within the page/post editor whenever you want to have the links available. When I add the shortcode to a post on my site, I currently see this:
The links work (double-check your links), but it doesn’t flow with the rest of the post or look particularly compelling to click on. Looks like it’s time for some CSS. Let’s start by getting the links centered and everything in one line.
.social-share-link-container {
width: 100%;
max-width: 400px;
margin: 100px auto;
}
.social-share-link-container ul {
list-style-type: none;
padding-left: 0px;
margin: 0px;
}
.social-share-link-container li {
display: inline-block;
}
Ok, things are coming along. Now for some title styles (keeping things simple, but making it stand out is key).
.social-share-link-container li.title {
padding: 5px 0;
text-transform: uppercase;
font-size: .8em;
padding-right: 5px;
font-weight: bold;
}
Now some basic link styles – again, I’m keeping things simple and just making the links into large, flat, grey buttons.
.social-share-link-container li.link {
background-color: #d8d8d8;
width: 100px;
text-align: center;
margin-right: 2px;
}
.social-share-link-container a {
color: white;
display: block;
width: 100%;
height: 100%;
padding: 5px 0;
}
Last but not least, let’s make sure that we add some hover/active styles for those links. I prefer to change the color of the button to that of the social network in question. Brand Colors is a pretty handy tool for finding the relevant colors for your networks of choice. Here are the styles for my social networks:
.social-share-link-container li.twitter a:hover,
.social-share-link-container li.twitter a:focus,
.social-share-link-container li.twitter a:active {
background-color: #55acee;
}
.social-share-link-container li.linkedin a:hover,
.social-share-link-container li.linkedin a:focus,
.social-share-link-container li.linkedin a:active {
background-color: #0077b5;
}
.social-share-link-container li.facebook a:hover,
.social-share-link-container li.facebook a:focus,
.social-share-link-container li.facebook a:active {
background-color: #3b5998;
}
Things are looking pretty good at this point – the links work, you can tell they’re clickable, the styles are simple but aesthetically pleasing. One final enhancement I like to add is to make the links open in a popup window versus opening in the same window. This is possible with some simple javascript/jQuery. First, a function to open a url in a centered popup window with a size appropriate to the device being used.
function socialPopup(url, size) {
// First, let's set the size of the popup. If the window is wider than 768px, set a fixed size:
var docWidth = $(window).width();
var docHeight = $(window).height();
if(docWidth >= 768) {
var popWidth = 600; var popHeight = 400;
}
// Otherwise, set the height and width to 80% of the window
else {
var popWidth = docWidth * .8;
var popHeight = docHeight * .8;
}
// Center the popup
var leftPos = (docWidth / 2) - (popWidth / 2);
var topPos = (docHeight / 2) - (popHeight / 2); window.open(url,"","menubar=no,toolbar=no,resizable=yes,scrollbars=yes,width=" + popWidth + ",height=" + popHeight + ",top=" + topPos + ",left=" + leftPos);
}
Then, simply attach this function to the links in your social sharing widget with some basic jQuery:
$('.social-share-link-container a').click(function(e) {
e.preventDefault();
socialPopup($(this).attr('href'));
});
And there you have it – a fully functional, simply enhanced widget which you can include at will in any post or page within your WordPress website.
Social Share Link Examples and Resources
I walked you through the process of building a Twitter share link, but you will likely want users to be able to share your content on other social networks. Here are examples and documentation links for several major networks:
Setting Basic Variables
global $post;
$postID = $post->ID;
$title = urlencode(get_the_title());
$url = urlencode(get_the_permalink());
$twitterName = 'heatherthedev';
$tags = 'wordpress,socialmedia';
$img = wp_get_attachment_image_src(get_post_thumbnail_id($postID));
$img = urlencode($img[0]);
$source = 'http://www.hweaver.com';
var twitterLink = "https://twitter.com/intent/tweet/?text=$title&url=$url&via=$twitterName&hashtags=$tags";
- Text – This is the body of the tweet. Not required.
- URL – The page URL. Not required.
- Via – Your twitter handle. Not required.
- Hashtags – A comma separated list of hashtags to append to the tweet. Not required.
var redditLink = "http://www.reddit.com/submit/?url=$url";
- URL – The URL you wish to share. Required
var pinLink = "https://www.pinterest.com/pin/create/button/?url=$url&media=$img&description=$title&hashtags=$tags";
- URL – The URL you want to share. Required.
- Media – The image for the pin. Not required.
- Description – A brief description of the pin (I use the post title). Not required.
var linkedLink = "https://www.linkedin.com/shareArticle?mini=true&url=$url&title=$title&source=$source";
- Mini – Must be set to true. Required.
- URL – The URL you want to share. Required.
- Title – The post title. Not required.
- Source – Your website URL. Not required.
Google+
var googleLink = "https://plus.google.com/share?url=$url";
- URL – The URL you want to share. Required.
var facebookLink = "https://www.facebook.com/sharer/sharer.php?u=$url";
- U – The page URL you want to share. Required.
While it takes a bit more time to build your own social share links, the payoffs are great – better control over design and responsive behavior and countless fewer HTTP requests on page load. Use this tutorial to start your widget, and then customize the CSS to fit your WordPress theme. Have questions? Ask in the comments below.
Download the code on Github
I've put together a full example you can download and implement right away